<Section>
A container element for grouping layout elements together. Each section has a header bar with title, icon button and collapsible content area for detailed UI elements.
Schema
#Sections are defined with type: ‘group’ under init.fields property.
const reducer = utReducer('react-example/sections', {
init: {
fields: {
customer: {
type: 'group',
child: {
first: {label: 'First Name',value: 'John'},
last: {label: 'Last Name'}
},
},
},
section: {
customer: false
}
},
})
init: {
fields: {
customer: {
type: 'group',
child: {
first: {label: 'First Name',value: 'John'},
last: {label: 'Last Name'}
},
},
},
section: {
customer: false
}
},
})
Section schemas are defined in init.fields object.
init: {
fields: {
<section id>: {
type: 'group',
isCollapse: false,
child: {
<field id>: {
...
},
...
},
},
},
},
fields: {
<section id>: {
type: 'group',
isCollapse: false,
child: {
<field id>: {
...
},
...
},
},
},
},
You can define multiple sections under init.fields with the following properties:
<section id>: string
Section id is a unique id for a section. In the above example, ‘customer’ is the section id.
type: string
type must be the value ‘group’ in order to recognize this is a section.
child: object
Layout
# <Section id='customer'
title='Customer Details'
isEditable = {true}
Icon = {FcCustomerSupport}
isSave = {true}
isCollapse = {true}
isCache = {true}
isPending = {true}
backgroundColor = 'WhiteSmoke'
>
title='Customer Details'
isEditable = {true}
Icon = {FcCustomerSupport}
isSave = {true}
isCollapse = {true}
isCache = {true}
isPending = {true}
backgroundColor = 'WhiteSmoke'
>
<Section> properties as follows:
id: String
A unique identifier for each <Section> element, the id must match with the field group id in state.
title: String / component
Section title displayed inside the title bar which can be a string or a React component.
isEditable: boolean
Control if the section fields are editable by users. If activated, the icon
will appear on the title bar.

Icon: component
react-icons component.
isSave: boolean
Control if the section fields can be saved. If activated, the save icon
will appear.

isCollapse: boolean
Control if the section fields can be expanded and collapsed. If activated, the collapse icon
will appear.

backgroundColor: string
Assign background color for the section header
Example
#The following example consists of a section ‘customer’ that is followed by a simple field called ‘preferences’.
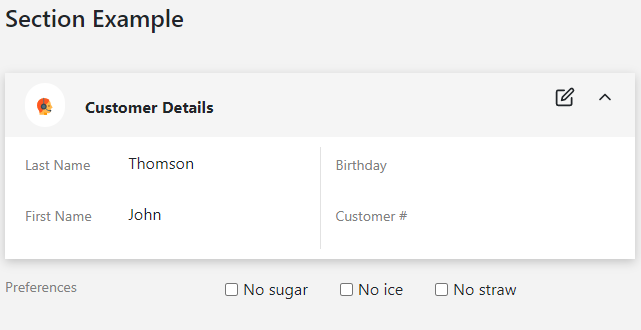
Visit example.u.team/section to try out the live examples.
Schema
# fields: {
customer: {
type: 'group',
child: {
first: {
label: 'First Name',
value: 'John',
cache: 'Johnny',
pending: 'Jonathan, Jonah'
},
last: {
label: 'Last Name',
value: 'Thomson',
},
customer: {
label: 'Customer #',
},
birthday: { },
},
},
preferences: {
type: "checkbox",
list: {c1 :'No sugar', c2 :'No ice', c3: 'No straw'}
},
},
customer: {
type: 'group',
child: {
first: {
label: 'First Name',
value: 'John',
cache: 'Johnny',
pending: 'Jonathan, Jonah'
},
last: {
label: 'Last Name',
value: 'Thomson',
},
customer: {
label: 'Customer #',
},
birthday: { },
},
},
preferences: {
type: "checkbox",
list: {c1 :'No sugar', c2 :'No ice', c3: 'No straw'}
},
},
Layout
# return (<>
<h4>Section Example</h4><br />
<Section id='customer' title='Customer Details'
Icon = {FcCustomerSupport}
isSave = {true}
isEditable = {true}
isCollapse = {true}
backgroundColor = 'WhiteSmoke'
>
<Row>
<Col>
<Field id='last' />
<Field id='first' />
</Col>
<Col>
<Field id='birthday' />
<Field id='customer' />
</Col>
</Row>
</Section>
<Field id="preferences" />
</>)
<h4>Section Example</h4><br />
<Section id='customer' title='Customer Details'
Icon = {FcCustomerSupport}
isSave = {true}
isEditable = {true}
isCollapse = {true}
backgroundColor = 'WhiteSmoke'
>
<Row>
<Col>
<Field id='last' />
<Field id='first' />
</Col>
<Col>
<Field id='birthday' />
<Field id='customer' />
</Col>
</Row>
</Section>
<Field id="preferences" />
</>)