Hello World
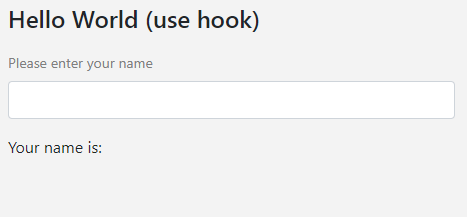
We will go through a simple Hello World example here. It consists of a text field name for user input. The name you are typing in will be displayed underneath.
Import @uteamjs Components
#First, two components utCreateElement and utReducer are required. Import from @uteamjs/react.
import { utCreateElement, utReducer } from '@uteamjs/react'
Fields Initialization
#Then we initialize the Reducer with a unique identifier react-example/hello-world-hook'.
const reducer = utReducer('react-example/hello-world-hook', {
init: {
fields: {
name: { label: 'Please enter your name' }
}}})
init: {
fields: {
name: { label: 'Please enter your name' }
}}})
In this example, the Reducer only initializes one object: fields.name. Which is a text field with an ID name for the user to input his name. If not specified, the default field type is text. For details of field properties refer to Field Properties.
Layout
#const layout = ({ _, Field }) =>
<>
<h4>Hello World (use hook)</h4>
<Field id='name' />
Your name is: {_.fields.name.value}
</>
<>
<h4>Hello World (use hook)</h4>
<Field id='name' />
Your name is: {_.fields.name.value}
</>
layout is a JSX component. Here, it contains:
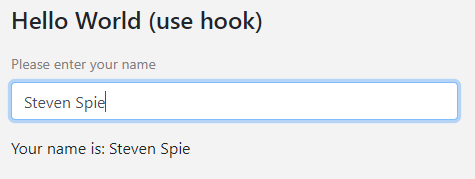
Create the Element
#export default utCreateElement({ reducer, layout })
Finally, utCreatElement() is the function to automate the connection of reducer to the element by injecting different properties into the layout components.