Low-Code Development
Low-code application development has become increasingly popular nowadays. Many of the solutions provide drag and drop visual design tools focused on end users to build applications around their business needs. Although it works in most simple cases, it is more difficult to apply in building complex enterprise applications. Update and maintenance is a big challenge for end user developed low-code software. Our solution is targeted at developers of all skills to enhance their development life cycle.
Our approach
#YAML
#React-Redux is by far one of the most popular frameworks for SPA single page web applications. We are focused on speeding up the development of existing React-Redux based applications which requires a lot of frontend form input processing and backend database services.
YAML is used to define the application flow and details. We carefully designed the syntax to be easily readable and meaningful to both end users and developers.
Example
#In this example, an input form with four different field types, there are 32 words to define the form. 23 out of 32 (more than 60%) words are the necessary items displayed on screen. Other keywords Fields, Column, tp, list, datepicker, select, textarea are being used to describe the field properties.
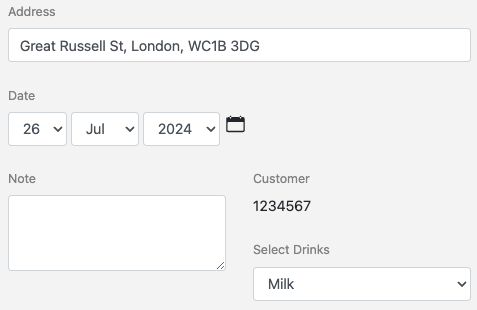
- Fields:
- Address: Great Russell St, London, WC1B 3DG
- Date: 26 July 2024
tp: datepicker
- Columns:
- Fields:
- Note:
tp: 'textarea'
- Fields:
- Customer: 1234567
readOnly: true
- Select Drinks:
tp: select
list:
- Milk
- Coffee
- Orange Juice
- Address: Great Russell St, London, WC1B 3DG
- Date: 26 July 2024
tp: datepicker
- Columns:
- Fields:
- Note:
tp: 'textarea'
- Fields:
- Customer: 1234567
readOnly: true
- Select Drinks:
tp: select
list:
- Milk
- Coffee
- Orange Juice
Using the Drag & Drop approach you need to enter at least 23 words, plus 40 more mouse moves, clicks and scrolls. Our approach using YAML only requires typing 32 words. Plus you can copy & paste the YAML easily to build your application.
The syntax is close to plain English which is minimal and effective to describe the behaviour of the application. Business users can also help to prepare the YAML as part of the application requirement and pass it to the developer for code generation and subsequent development.
Code Generated
#Based on the YAML, there are 60 lines of codes generated. The generated code is a standard JSX React component using the @uteamjs/react framework we provided. There is only a single JSX file generated without any boilerplates. The file is readable and also most importantly easy to maintain.
import { utCreateElement, utform, utReducer } from '@uteamjs/react'
import { Col, Row } from 'react-bootstrap'
const reducer = utReducer('yaml-example/fields', {
init: {
fields: {
address: {
label: "Address",
value: "Great Russell St, London, WC1B 3DG"
},
date: {
type: "datepicker",
label: "Date",
value: "26 July 2024"
},
note: {
type: "textarea",
label: "Note"
},
customer: {
label: "Customer",
value: 1234567
},
selectDrinks: {
type: "select",
list: {
milk: "Milk",
coffee: "Coffee",
orangejuice: "Orange Juice"
},
label: "Select Drinks"
}
},
},
})
class layout extends utform {
render = () => {
const { Field } = this
return (<>
<Field id="address" />
<Field id="date" />
<Row>
<Col>
<Field id="note" no={2} />
</Col>
<Col>
<Field id="customer" readOnly="true" no={2} />
<Field id="selectDrinks" no={2} />
</Col>
</Row>
</>)
}
}
export default utCreateElement({ reducer, layout })
import { Col, Row } from 'react-bootstrap'
const reducer = utReducer('yaml-example/fields', {
init: {
fields: {
address: {
label: "Address",
value: "Great Russell St, London, WC1B 3DG"
},
date: {
type: "datepicker",
label: "Date",
value: "26 July 2024"
},
note: {
type: "textarea",
label: "Note"
},
customer: {
label: "Customer",
value: 1234567
},
selectDrinks: {
type: "select",
list: {
milk: "Milk",
coffee: "Coffee",
orangejuice: "Orange Juice"
},
label: "Select Drinks"
}
},
},
})
class layout extends utform {
render = () => {
const { Field } = this
return (<>
<Field id="address" />
<Field id="date" />
<Row>
<Col>
<Field id="note" no={2} />
</Col>
<Col>
<Field id="customer" readOnly="true" no={2} />
<Field id="selectDrinks" no={2} />
</Col>
</Row>
</>)
}
}
export default utCreateElement({ reducer, layout })
The generated code is discussed in the next section.