<Grid>
Grid table Element based on AG Grid React UI.
Schema
#Grid columns and rows are initialized in the reducer under init.fields property.
const reducer = utReducer('react-example/grid', {
init: {
columns: [
{field: "orderNo", headerName: "Order No." },
{field: "value", headerName: "Order Value" },
],
rows: [
{ orderNo: "MO20240700123", value: 210 },
{ orderNo: "MO20240800246", value: 350 },
{ orderNo: "MO20260200369", value: 110 }
]
}
})
init: {
columns: [
{field: "orderNo", headerName: "Order No." },
{field: "value", headerName: "Order Value" },
],
rows: [
{ orderNo: "MO20240700123", value: 210 },
{ orderNo: "MO20240800246", value: 350 },
{ orderNo: "MO20260200369", value: 110 }
]
}
})
Columns
#Major attributes under the columns property:
field: string
A unique id for the column
headerName: string
Caption for the column
width: string
Width of column in pixels or %
filter: string
When assigned 'agTextColumnFilter', a filter icon will appear on the corresponding column header.
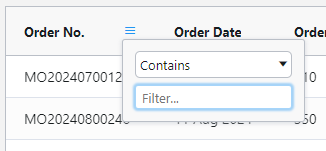
editable: boolean
Control column fields can be edited or not. Input either true or false.
Refer to AG Grid/Column Definitions for more information.
Rows
#Under the rows property is the array of row records in the following format:
rows: [
{ field 1: value1, field2: value1, ... },
...
]
{ field 1: value1, field2: value1, ... },
...
]
field 1, field 2, …
Field ID in column 1, 2 ...
value 1, value 2, ..,
Data value in column 1, 2 ...
Layout
#class layout extends utform {
render = () => {
const { Field, Grid } = this
return (<>
<h4>Simple Grid</h4><br />
...
<Grid domLayout='autoHeight' />
</>)
}
}
render = () => {
const { Field, Grid } = this
return (<>
<h4>Simple Grid</h4><br />
...
<Grid domLayout='autoHeight' />
</>)
}
}
<Grid> properties as follows:
domLayout
It specifies the layout of the grid.
autoHeight - fit the rows so that no vertical scrollbar is needed
print - grid renders all rows and columns without scrollbar
Refer to AG Grid/Grid Properties for more information.
Example
#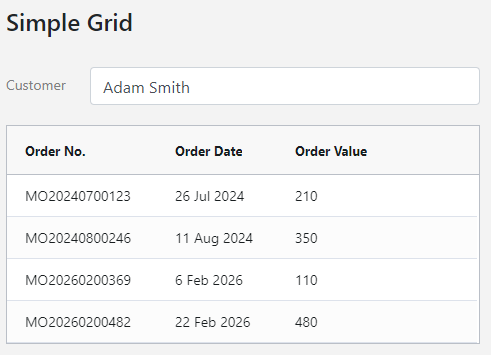
Visit example.u.team/grid to try out the live examples.
Field
#A non-grid field ‘customer’ is added in this example.
const reducer = utReducer('react-example/grid', {
init: {
isEdit: true,
fields: {
customer: {
label: "Customer",
value: "Adam Smith",
},
},
// Grid column data here
columns: [ … ],
rows: [ … ],
}
})
init: {
isEdit: true,
fields: {
customer: {
label: "Customer",
value: "Adam Smith",
},
},
// Grid column data here
columns: [ … ],
rows: [ … ],
}
})
Columns
#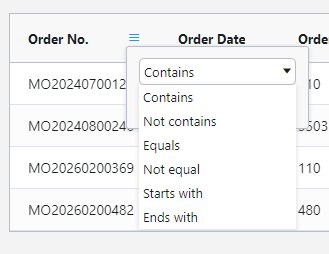
columns: [
{
field: "orderNo",
headerName: "Order No.",
width: "150%",
filter: 'agTextColumnFilter'
},
{
field: "date",
headerName: "Order Date",
width: 120
},
{
field: "value",
headerName: "Order Value",
editable: true
}
],
{
field: "orderNo",
headerName: "Order No.",
width: "150%",
filter: 'agTextColumnFilter'
},
{
field: "date",
headerName: "Order Date",
width: 120
},
{
field: "value",
headerName: "Order Value",
editable: true
}
],
Rows
# rows: [
{ orderNo: "MO20240700123", date: "26 Jul 2024", value: 210 },
{ orderNo: "MO20240800246", date: "11 Aug 2024", value: 350 },
{ orderNo: "MO20260200369", date: "6 Feb 2026", value: 110 },
{ orderNo: "MO20260200482", date: "22 Feb 2026", value: 480 },
],
{ orderNo: "MO20240700123", date: "26 Jul 2024", value: 210 },
{ orderNo: "MO20240800246", date: "11 Aug 2024", value: 350 },
{ orderNo: "MO20260200369", date: "6 Feb 2026", value: 110 },
{ orderNo: "MO20260200482", date: "22 Feb 2026", value: 480 },
],
Layout
#class layout extends utform {
render = () => {
const { Field, Grid } = this
return (<>
<h4>Simple Grid</h4><br />
<Field id="customer" />
<Grid domLayout='autoHeight' />
</>)
}
}
render = () => {
const { Field, Grid } = this
return (<>
<h4>Simple Grid</h4><br />
<Field id="customer" />
<Grid domLayout='autoHeight' />
</>)
}
}