Extension
There are two possible types of extension that can be applied to the framework for Hook components:
<Field> types extension
#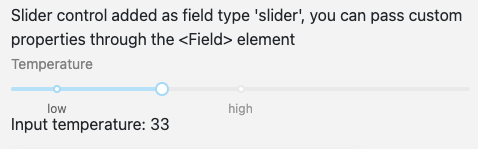
Visit example.u.team/extension to try out the live examples.
import Slider from 'rc-slider'
const mySlider = param => {
const { value, isRead, onChange, props } = param
props.disabled = isRead || props.disabled
return <Slider {...props} value={value}
onChange={value => onChange({ target: { value } })} />}
const myExtension = _this => {
_this.customfield = { ..._this.customfield, slider: mySlider }
}
// Replace utCreateElement
const myCreateElement = props => {
props.param = { ...props.param, extend: myExtension }
return utCreateElement(props)}
export default myCreateElement({ reducer, layout })
const mySlider = param => {
const { value, isRead, onChange, props } = param
props.disabled = isRead || props.disabled
return <Slider {...props} value={value}
onChange={value => onChange({ target: { value } })} />}
const myExtension = _this => {
_this.customfield = { ..._this.customfield, slider: mySlider }
}
// Replace utCreateElement
const myCreateElement = props => {
props.param = { ...props.param, extend: myExtension }
return utCreateElement(props)}
export default myCreateElement({ reducer, layout })
Schema
#import Slider from 'rc-slider'
const reducer = utReducer('react-example/extend-userHook', {
init: {
...
fields: {
...
temperature: {type: 'slider', value: 28}
},}
})
const reducer = utReducer('react-example/extend-userHook', {
init: {
...
fields: {
...
temperature: {type: 'slider', value: 28}
},}
})
Layout
#const layout = ({ _, Field, Memo }) => <>
Slider control added as field type 'slider',
you can pass custom properties through the <Field> element
<Field id='temperature' marks={{
10: {label: 'low'}, 50: {label: 'high'}}} />
<p>Input temperature: {_.fields.temperature.value}</p>
</>
Slider control added as field type 'slider',
you can pass custom properties through the <Field> element
<Field id='temperature' marks={{
10: {label: 'low'}, 50: {label: 'high'}}} />
<p>Input temperature: {_.fields.temperature.value}</p>
</>
Component Extension
#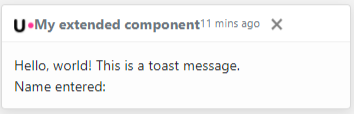
import { Toast } from 'react-bootstrap'
const myMemo = _this => ({ title }) => {
const { _ } = _this.props.init
return <Toast>
<Toast.Header>
<img src="/assets/icons/favicon.png"
width='20px' className="rounded me-2" alt="" />
<strong className="me-auto">{title}</strong>
<small>11 mins ago</small>
</Toast.Header>
<Toast.Body>Hello, world! This is a toast message.
<div>Name entered: {_.fields.name.value}</div>
</Toast.Body>
</Toast>
}
const myMemo = _this => ({ title }) => {
const { _ } = _this.props.init
return <Toast>
<Toast.Header>
<img src="/assets/icons/favicon.png"
width='20px' className="rounded me-2" alt="" />
<strong className="me-auto">{title}</strong>
<small>11 mins ago</small>
</Toast.Header>
<Toast.Body>Hello, world! This is a toast message.
<div>Name entered: {_.fields.name.value}</div>
</Toast.Body>
</Toast>
}
const myExtension = _this => {
_this.Memo = myMemo(_this)
return { Memo: _this.Memo }
}
const myCreateElement = props => {
props.param = { ...props.param, extend: myExtension }
return utCreateElement(props)}
export default myCreateElement({ reducer, layout })
_this.Memo = myMemo(_this)
return { Memo: _this.Memo }
}
const myCreateElement = props => {
props.param = { ...props.param, extend: myExtension }
return utCreateElement(props)}
export default myCreateElement({ reducer, layout })
Layout
#const layout = ({ _, Field, Memo }) => <>
...
<Memo title='My extended component' />
</>
...
<Memo title='My extended component' />
</>