Get started
Requirements
#To run the uteam CLI (Command Line Interface) you need to install Node.js at version 12 with NPM version 7 or above. If you don't have it installed, download Node.js from https://nodejs.org/en/download/, and follow the installation steps for your computer.
Installation
#uteam CLI
#The uteam CLI (Command Line Interface) allows you to start, build, and export your application. Use the npm global option for installation.
$ npm install -g uteam
To get a list of the available CLI commands, run the following command inside your project directory. Please make sure your computer can access the internet when using uteam cli.
$ uteam -h
Create your first application
#Create a project folder for all @uteamjs application (optional):
$ mkdir <project_folder>
Change to your project folder and run the following commands in your terminal:
$ cd <project_folder>
Create your first application ‘tutorial-react’ with one ‘examples’ package:
$ uteam create -a tutorial-react -p get-started
The following directory will be created under <project_folder>:
//tutorial-react/ node_modules/ @uteamjs/react packages/ get-started/ ... app.yaml main/ node_modules/ get-started config/ ... src/ index.js package.json
You can add packages under the ‘tutorial-react’ application. Each package is a module with a tree menu and corresponding pages. The package is installed as a link in node_modules. ‘main’ is a special package which provides the main entry point for the application.
Generate code from app.yaml
#Change to get-started folder:
$ cd ./tutorial-react/packages/get-started
Open the app.yaml to edit (optional):
header:
name: Get Started # Package Name displayed in main-menu bar
template: "@uteamjs/template/react-redux"
#individual: true # Generate individual file
src: src # Output directory for js file
# Index Template
module: get-started
index: index.js # Index file name
routeName: routeGetStarted
routePath: get-started
defaultPath: introduction
# Tree Menu for application
menu:
- Introduction: introduction
- Pages:
- 'Hello World': helloworld
# First component page
introduction:
#- generate
- h1: Introduction
- h4: Welcome to u.team
- p: |
To modify this page,
edit the app.yaml in corresponding packages
# Second component page
helloworld:
- h1: Hello World
- Fields:
- Age
- Name Please: Peter
id: name
- div: You name is %name%
name: Get Started # Package Name displayed in main-menu bar
template: "@uteamjs/template/react-redux"
#individual: true # Generate individual file
src: src # Output directory for js file
# Index Template
module: get-started
index: index.js # Index file name
routeName: routeGetStarted
routePath: get-started
defaultPath: introduction
# Tree Menu for application
menu:
- Introduction: introduction
- Pages:
- 'Hello World': helloworld
# First component page
introduction:
#- generate
- h1: Introduction
- h4: Welcome to u.team
- p: |
To modify this page,
edit the app.yaml in corresponding packages
# Second component page
helloworld:
- h1: Hello World
- Fields:
- Age
- Name Please: Peter
id: name
- div: You name is %name%
Assume you're still in ...packages/get-started folder, go ahead to generate the JSX code:
$ uteam generate
Three js files under ‘get-started’ will be created.
//tutorial-react/ ... packages/ main/ src/ index.js get-started/ src/ helloworld.js introduction.js index.js
The index.js file under main/src will be modified. routeExamples is imported and pasted in two lines.
// 1. Import generated packages
import { routeGetStarted } from '../../get-started'
/** insert import */
// 2. Put all imported packages into a array
const modules = [
routeGetStarted,
/** insert module */
]
import { routeGetStarted } from '../../get-started'
/** insert import */
// 2. Put all imported packages into a array
const modules = [
routeGetStarted,
/** insert module */
]
Note: DO NOT remove the comment lines /** insert import */ and /** insert module */ which are insertion points for additional packages.
Test run your applications
#Change to ...packages/main folder, assuming you’re staying in examples folder:
$ cd ../main
Start the webpack development server:
$ npm start
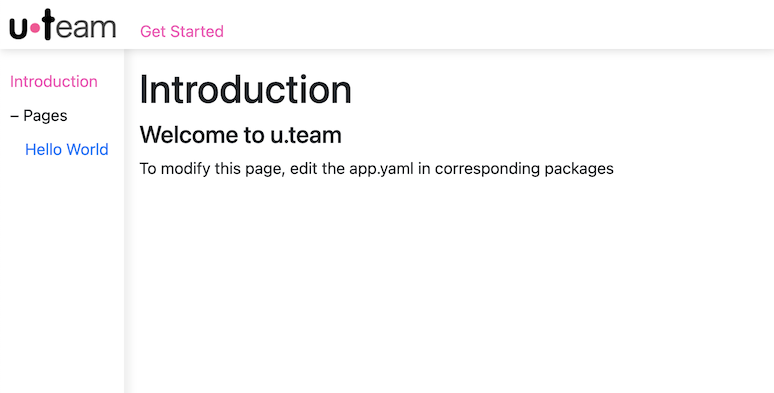
Add new packages
#You can create additional packages to the same application using @uteamjs/template or your custom template.
YAML examples
#Open a new terminal, then change to your tutorial-react folder:
$ cd <project_folder>/tutorial-react
Create your yaml-examples from template yaml-examples:
$ uteam create -p yaml-examples -t yaml-examples -g
The -g option is to run generate after each package created. You can skip to run uteam generate manually as shown in the above example.
Assuming your webpack development server still running, open your browser with URL http://localhost:3000, you will see there are two packages on the top menu bar, then click the Yaml Examples link:
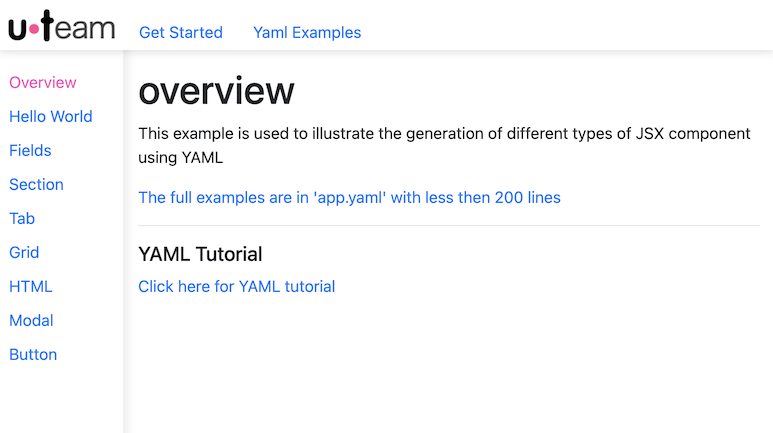
You can play around with .../tutorial-react/packages/yaml-examples/app.yaml, then regenerate the code again.
YAML CRUD
#A full example with the following features:
These are more advanced examples to illustrate CRUD operation. If you want to try it out, go back to your project folder.
$ cd <project_folder>/tutorial-react
Create your crud from template yaml-crud:
$ uteam create -p crud -t yaml-crud -g
Open your browser with URL http://localhost:3000, you will see there are three packages on the top menu bar, then click the Crud link:
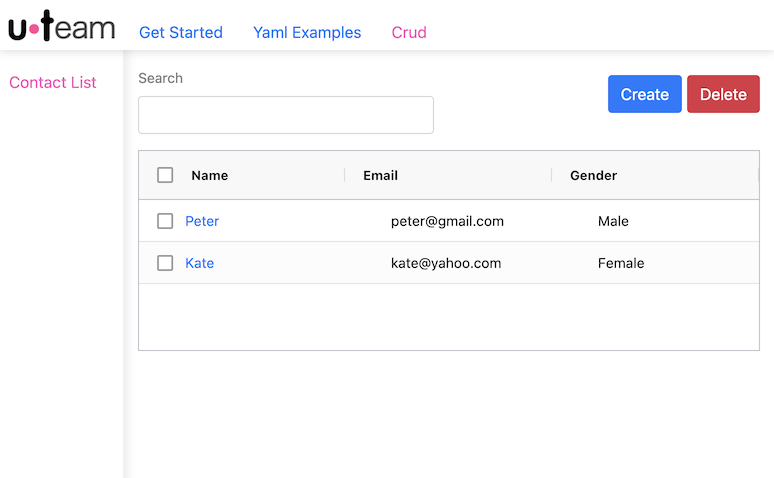
Integrate with backend API
#This example is based on the above YAML CRUD plus Node.js backend API integration.
Create React Frontend
#Go back to your application folder.
$ cd <project_folder>/tutorial-react
Create the crud-api package from template yaml-crud-api:
$ uteam create -p crud-api -t yaml-crud-api -g
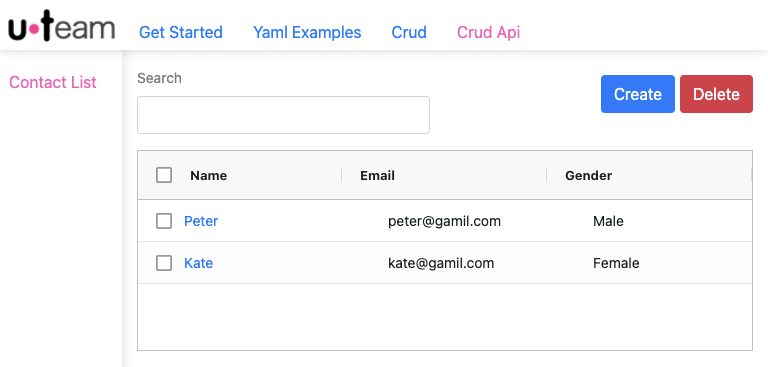
Right now, when you try to perform any CRUD action, there will be fetching errors because the backend server is not available. We need to create the Node.js backend in the following sections.
Create Node.js Backend
#Change to your project folders (not application folder) again:
$ cd <project_folder>
Create your backend application ‘tutorial-node’ using template ‘node-application’:
$ uteam create -a tutorial-node -t node-application
Similar to the front end application, the follow folder is created:
//tutorial-node/ ... packages/ main/ config.json package.json server.js
Then change to the newly created application folder:
$ cd tutorial-node
Add the package ‘crud-api’ using template ‘node-crud-api’:
$ uteam create -p crud-api -t node-crud-api
Package ‘crud-api` added:
//tutorial-node/ ... packages/ main/ ... crud-api/ contact.js detail.js package.json
contact.js
#There are three APIs load, add and delete mapped to the corresponding front end actions in <project_folder>/tutorial-react/packages/crud-api/src/contact.js:
const ut = require('@uteamjs/node')
exports.load = ut.sqlseries((db, data) => [
db.query('select * from contact', rows => {
rows.forEach(t => t.gender = ut.capitalize(t.gender))
data.rows = rows
})
])
exports.add = ut.sqlseries((db, payload) => [
db.updateInsert(null, payload.id, 'contact', payload)
])
exports.delete = ut.sqlseries((db, payload) => [
db.query(`delete from contact where id in (#123;payload.toString()})`)
])
exports.load = ut.sqlseries((db, data) => [
db.query('select * from contact', rows => {
rows.forEach(t => t.gender = ut.capitalize(t.gender))
data.rows = rows
})
])
exports.add = ut.sqlseries((db, payload) => [
db.updateInsert(null, payload.id, 'contact', payload)
])
exports.delete = ut.sqlseries((db, payload) => [
db.query(`delete from contact where id in (#123;payload.toString()})`)
])
detail.js
#The load API mapped to the front end actions in <project_folder>/tutorial-react/packages/crud-api/src/detail.js:
const ut = require('@uteamjs/node')
exports.load = ut.sqlseries((db, payload) => [
db.query(`select * from contact where id='#123;payload.id}'`, rows => {
payload.data = rows[0]
payload.data.gender = payload.data.gender.toLowerCase()
})
]
exports.load = ut.sqlseries((db, payload) => [
db.query(`select * from contact where id='#123;payload.id}'`, rows => {
payload.data = rows[0]
payload.data.gender = payload.data.gender.toLowerCase()
})
]
Change to the main package folder:
$ cd packages/main
Start the backend server:
$ node server
When you test run the crud-api package, the frontend CRUD action will be posted back to the backend sqlite database. Since the database is created in memory, it will be reset each time you restart the server.